Creating a dynamic table is an essential part of any web application, and React JS provides an excellent framework for doing so. In this tutorial, we will walk you through the process on how to create dynamic table in React JS, step by step.
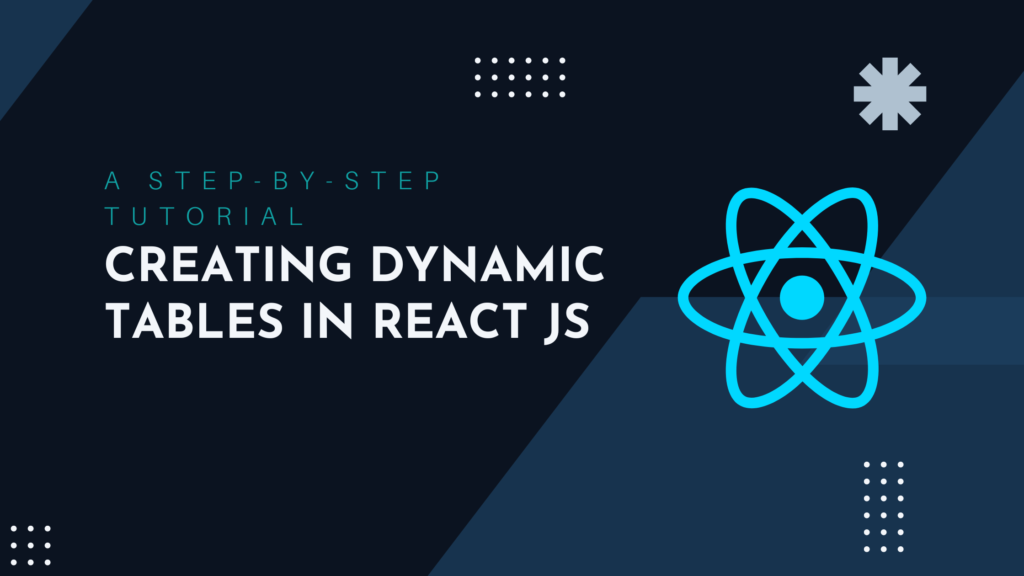
Table of Contents
How to create dynamic table in React JS
Before we get started, let’s define what a dynamic table is. A dynamic table is a table that can be easily updated and modified based on user input or data changes. In other words, a dynamic table can be created and modified on the fly.
Step 1: Setting up the project
To create a dynamic table in React JS, we first need to set up a React project. We will use create-react-app to create a new project. Open your terminal and type the following command:
npx create-react-app dynamic-table
This will create a new React project with the name “dynamic-table.”
Step 2: Creating the table component
Next, let’s create a new component for our table. In the src folder of your project, create a new file called Table.js. Inside this file, we will create a new React component for our table.
import React from 'react';
class Table extends React.Component {
constructor(props) {
super(props);
this.state = {
data: [
{ id: 1, name: 'John Doe', age: 25 },
{ id: 2, name: 'Jane Doe', age: 30 },
{ id: 3, name: 'Bob Smith', age: 35 }
]
}
}
render() {
return (
<div>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
{this.state.data.map((row) => (
<tr key={row.id}>
<td>{row.id}</td>
<td>{row.name}</td>
<td>{row.age}</td>
</tr>
))}
</tbody>
</table>
</div>
);
}
}
export default Table;
In this code, we have defined a new class called Table that extends React.Component. We have also defined a constructor that sets the initial state of our component, which is an array of data. This data will be used to populate our table.
Inside the render() method, we have created a table with three columns: ID, Name, and Age. We have also used the map() method to loop through the data array and create a new row for each item in the array.
Note that we have included a key property for each row. This is important for React to keep track of each item in the list and to optimize the rendering of the table.
Step 3: Rendering the table component
Now that we have created our Table component, let’s render it in our App component. In the src folder of your project, open the App.js file and update it with the following code:
import React from 'react';
import Table from './Table';
class App extends React.Component {
render() {
return (
<div>
<h1>Dynamic Table Example</h1>
<Table />
</div>
);
}
}
export default App;
In this code, we have imported our Table component and rendered it inside the App component. We have also added a heading to our page to display the title of our example.
Step 4: Adding dynamic data
Now that we have our basic table set up, let us focus on main part, how to create dynamic table in React JSS. For this, let’s add some dynamic data to it. In a real-world application, the data for our table would come from an API or a database. For the purpose of this tutorial, we will add a button that will allow us to add a new row of data to our table.
First, let’s update our Table component to include a button and a method for adding new data:
import React from 'react';
class Table extends React.Component {
constructor(props) {
super(props);
this.state = {
data: [
{ id: 1, name: 'John Doe', age: 25 },
{ id: 2, name: 'Jane Doe', age: 30 },
{ id: 3, name: 'Bob Smith', age: 35 }
]
}
this.addRow = this.addRow.bind(this);
}
addRow() {
const newData = { id: 4, name: 'New Person', age: 20 };
this.setState({ data: [...this.state.data, newData] });
}
render() {
return (
<div>
<button onClick={this.addRow}>Add Row</button>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
{this.state.data.map((row) => (
<tr key={row.id}>
<td>{row.id}</td>
<td>{row.name}</td>
<td>{row.age}</td>
</tr>
))}
</tbody>
</table>
</div>
);
}
}
export default Table;
In this code, we have added a new button element to our table and a method called addRow() that will add a new row of data to our table when called. We have also bound the addRow() method to the component’s this keyword to ensure that it has access to the correct context.
Inside the addRow() method, we have created a new object called newData with an id, name, and age property. We then use the setState() method to update the component’s state with the new data using the spread operator (…).
Finally, we have updated the render() method to include the new button element and to use the updated data array when rendering the table.
Step 5: Styling the table
Now that our dynamic table is working, let’s add some basic styling to make it look better. In the src folder of your project, create a new file called Table.css and add the following CSS code:
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
border-bottom: 1px solid #ddd;
}
th {
background-color: #f2f2f2;
color: #333;
}
This code will style our table to have a collapsed border, left-aligned text, and a gray background color for the header row.
Next, import the Table.css file in our Table.js component by adding the following code at the top of the file:
import React from 'react';
import './Table.css';
// rest of the code
Now, when you run your application, you should see your dynamic table with the new styling applied.
Conclusion
In this tutorial, we have shown you how to create a dynamic table in React JS step by step. We started by setting up a new React project, creating a new component for our table, rendering the table component in our main App component, adding dynamic data to our table, and finally, styling our table.
With the knowledge gained from this tutorial, you should now be able to create dynamic tables in your own React projects. Remember that there are many different ways to create dynamic tables in React, and this tutorial is just one example. You can customize your table to include more data, add additional features like sorting and filtering, and style it to fit your project’s specific needs.
When working with dynamic tables in React, it is important to keep in mind the performance implications of rendering large datasets. If your table contains a large amount of data, consider using pagination or virtualization to only render the data that is visible on the screen at any given time.
We hope this tutorial has been helpful in getting you started with creating dynamic tables in React JS. If you have any questions or comments, feel free to leave them below. Happy coding!
To read more such articles on React JS. Click Here